4. Python의 데이터 타입 (data type) : 6개 python의 built-in data type (이미 정의되있는 데이터 타입) Numeric (숫자) (int, float, complex) Sequence (순서가 있음) - list, tuple, range Text Sequence Type (str) : 문자열 Mapping : dictionary Bool 4-1. Numeric Data Type (숫자형) 다른 언어는 정수와 실수로 구분, 파이썬은 구분 안하지만 처리 시에는 floating 실수형으로 처리 int (정수) float (실수) complex (복소수) a = 100 # 정수 b = 3.14159265358979 # 실수 c = 1 + 2j # 복소수 d = 0o34 # ..
2020년 7월부터 멀티캠퍼스 융복합프로젝트형 AI 서비스 개발을 수강하며, 포스타입 블로그에 정리했던 내용들을 가져옵니다. Day01_Python - Introduction 200714 1. 주석 python의 주석은 1줄 주석은 => 여러 줄 주석은 """ """, ''' ''' 여러 블록 + ctrl _ / => 한꺼번에 주석 처리 2. Python의 keyword import keyword print (keyword.kwlist) # ['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', '..
20201115 윈도우 환경 mediapipe hand tracking import os import cv2 import mediapipe as mp mp_drawing = mp.solutions.drawing_utils mp_hands = mp.solutions.hands def track(input_data_path): # For webcam input: hands = mp_hands.Hands( min_detection_confidence=0.7, min_tracking_confidence=0.5) # cap = cv2.VideoCapture(0) => default: webcam input cap = cv2.VideoCapture(input_data_path) # cap = cv2.VideoCap..
programmers.co.kr/learn/courses/30/lessons/42626 코딩테스트 연습 - 더 맵게 매운 것을 좋아하는 Leo는 모든 음식의 스코빌 지수를 K 이상으로 만들고 싶습니다. 모든 음식의 스코빌 지수를 K 이상으로 만들기 위해 Leo는 스코빌 지수가 가장 낮은 두 개의 음식을 아래와 같 programmers.co.kr import heapq # 최소 힙 자료구조 h = [1, 2, 3] heapq.heapify(h) print(heapq.heappop(h)) # 1 - 최소값 반환 heapq.heappush(h, 0) # 입력 후 가장 작은 값이 앞에 오게 자동 정렬 print(heapq.heappop(h)) # 0 - 최소값 반환 import heapq def solution..
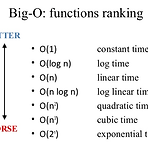
List 배열의 크기가 증가하거나 index 값이 작은 부분의 삽입, 삭제가 비효율적 append() : O(1) pop last : O(1) insert : O(n) delete : O(n) collections.deque append(), appendleft() : O(1) popleft(), pop() : O(1) from collections import deque dq = deque([1, 2, 3]) dq.append(n) dq.popleft()
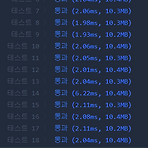
programmers.co.kr/learn/courses/30/lessons/12913 코딩테스트 연습 - 땅따먹기 땅따먹기 게임을 하려고 합니다. 땅따먹기 게임의 땅(land)은 총 N행 4열로 이루어져 있고, 모든 칸에는 점수가 쓰여 있습니다. 1행부터 땅을 밟으며 한 행씩 내려올 때, 각 행의 4칸 중 한 칸만 밟 programmers.co.kr 완전탐색하면 당연히 시간 초과 나고 한 행 씩 내려갈 수록 값은 커지며, 그 전 행의 값들 중 같은 열이 아니면서 가장 큰 값을 더한 값만 계산하며 배열의 값을 갱신해주면 된다. 예시> [[1,2,3,5],[5,6,7,8],[4,3,2,1]] 1 2 3 5 10 (5 + 5) 11 (5 + 6) 12 (5 + 7) 11 (3 + 8) 16 (12 + 4) ..
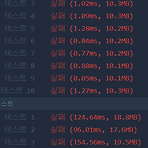
programmers.co.kr/learn/courses/30/lessons/42584 코딩테스트 연습 - 주식가격 초 단위로 기록된 주식가격이 담긴 배열 prices가 매개변수로 주어질 때, 가격이 떨어지지 않은 기간은 몇 초인지를 return 하도록 solution 함수를 완성하세요. 제한사항 prices의 각 가격은 1 이상 10,00 programmers.co.kr 분명 전에 풀었던 문제인데 못풀었다?!?!?! 바로 다음 초의 주식이 작아지더라도, 1초동안은 유지한걸로 치는걸 감안해서 작성했더니 오답이 나온다. def solution(prices): answer = [0] * len(prices) for i in range(len(prices)): cnt = 0 for j in range(i+1,..
www.acmicpc.net/problem/16769 16769번: Mixing Milk The first line of the input file contains two space-separated integers: the capacity $c_1$ of the first bucket, and the amount of milk $m_1$ in the first bucket. Both $c_1$ and $m_1$ are positive and at most 1 billion, with $c_1 \geq m_1$. The second and t www.acmicpc.net modular 연산은 정말 최고야 bucket = [0]*3 amount = [0]*3 for i in range(3): bucket[..